Module Boundaries
Every directory with an index.ts is a module. index.ts exports those files that should be accessible from the outside, i.e. it exposes the public API of the module.
In the screenshot below, you see an index.ts, which exposes the holidays-facade.service.ts, but encapsulates the internal.service.ts.
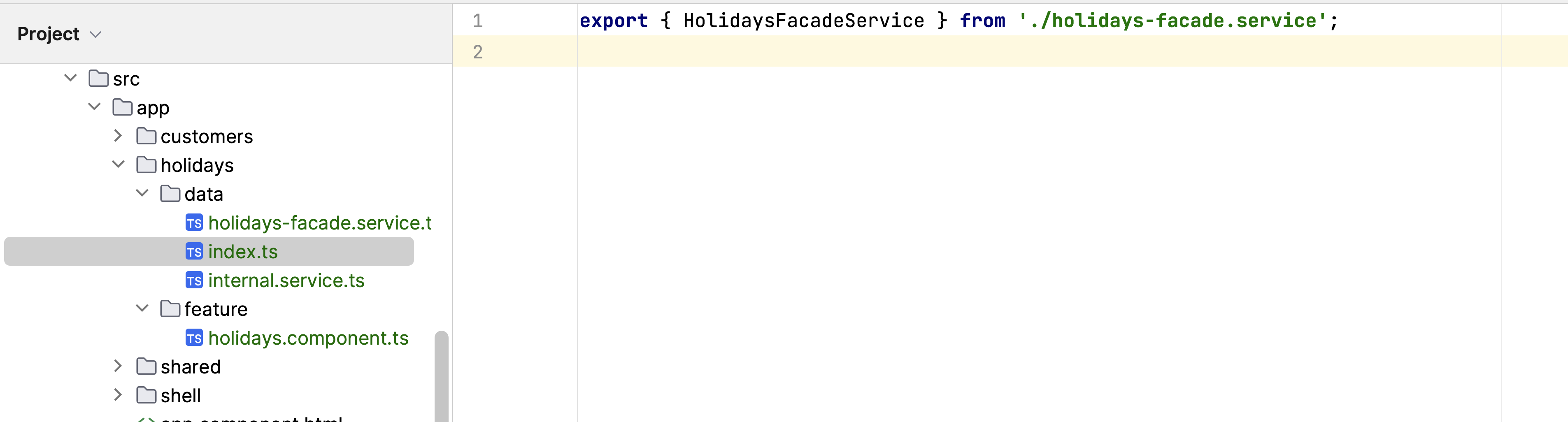
Every file outside of that directory (module) now gets a linting error when it imports the internal.service.ts.
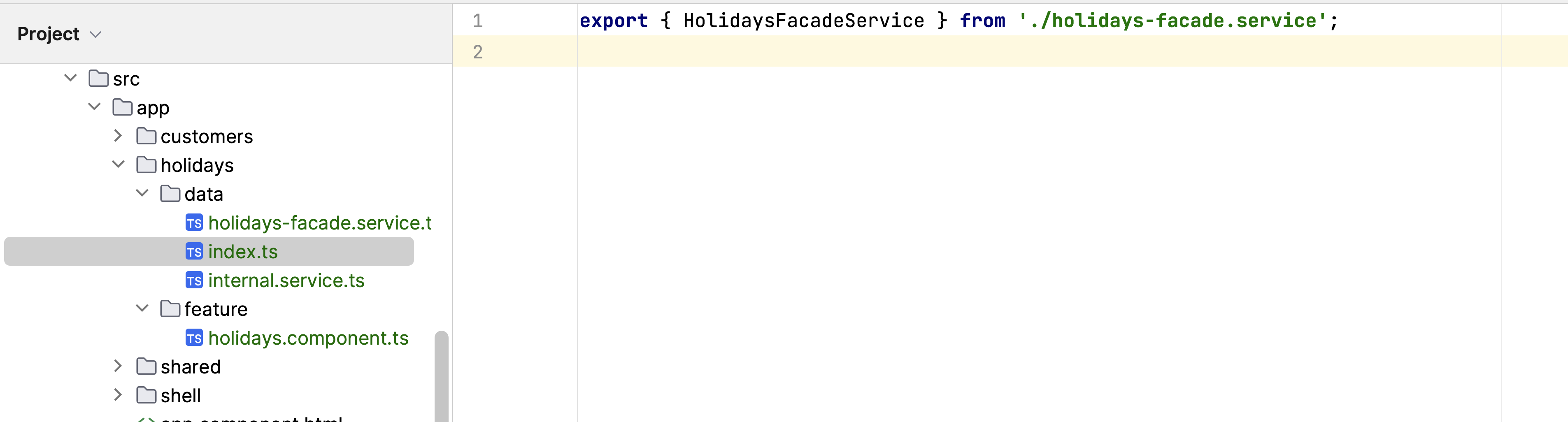